In this article, I am going to discuss the Data Types in C# with examples. Please read our previous article where we discuss the Console class Methods and Properties in C# before proceeding to this article. As a developer, it is very important to understand the Data Type in C#. This is because you need to decide which data type to use for a specific type of values. As part of this article, we are going to discuss the following pointers related to C# data type in detail.
- Why we need data types in C#?
- What is a data type in C#?
- Different types of Data type in C#.
- What is Value Data Type in C#?
- What is Reference Data Type in C#?
- Examples using Built-in Data Types in C#.
- What is Pointer Type?
- Examples using Escape Sequences in C#.
Why we need data types in C#?
The Datatypes in C# are basically used to store the data temporarily in the computer through a program. In the real world, we have different types of data like integer, floating-point, character, string, etc. To store all these different kinds of data in a program to perform business-related operations, we need the data types.
What is a data type in C#?
The Datatypes are something which gives information about
- Size of the memory location.
- The range of data that can be stored inside that memory location
- Possible legal operations which can be performed on that memory location.
- What types of results come out from an expression when these types are used inside that expression.
The keyword which gives all the above information is called the data type.
What are the different types of Data type available in C#?
A data type in C# specifies the type of data that a variable can store such as integer, floating, character, string, etc. The following diagram shows the different types of data types available in C#.
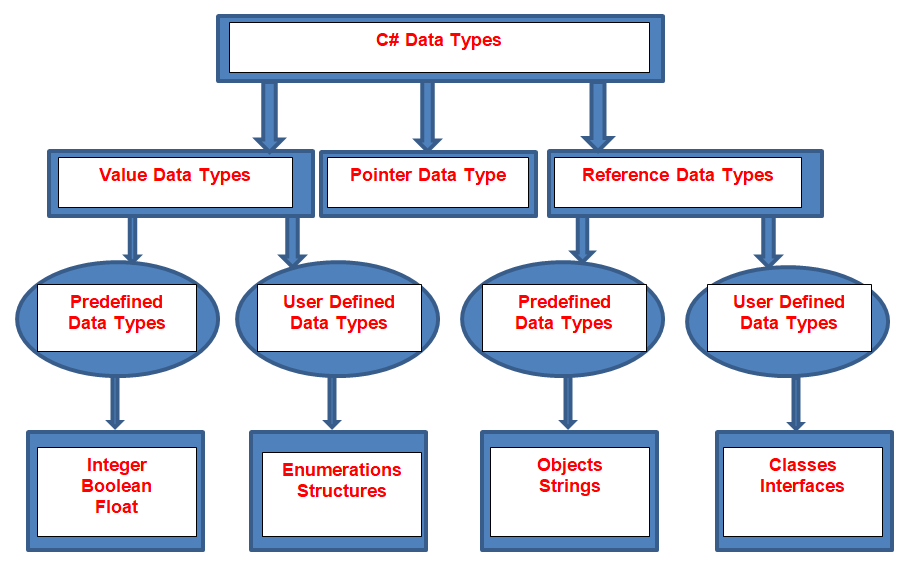
There are 3 types of data types available in the C# language.
- Value Data Type
- Reference Data Type
- Pointer Data Type
Let us discuss each of these data types in detail
What is Value Data Type in C#?
The data types which stores the value directly called as the Value Data Type. They are derived from the class System.ValueType. The examples are int, char, and float which stores numbers, alphabets, and floating-point numbers respectively. The value data types in C# again classified into two types are as follows.
- Predefined Data Types – Example includes Integer, Boolean, Float, etc.
- User-defined Data Types – Example includes Structure, Enumerations, etc.
Based on the Operating system (32 or 64-bit), the size of the memory of the data types may change. If you want to know the actual size of a type or a variable on a particular operating system, then you can make use of the sizeof method.
Let’s understand this with an example. The following example gets the size of int type on any platform.
- namespace FirstProgram
- {
- class Program
- {
- static void Main(string[] args)
- {
- Console.WriteLine("Size of int: {0}", sizeof(int));
- Console.ReadKey();
- }
- }
- }
When we execute the above code, it gives the following output:

What is Reference Data Type in C#?
The data type which is used to store the reference of a variable is called as Reference Data Types. In other words, we can say that the reference types do not store the actual data stored in a variable, rather they store the reference to the variables. We will discuss this concept in a later article.
Again, the Reference Data Types are categorized into 2 types. They are as follows.
- Predefined Types – Examples include Objects, String and dynamic.
- User-defined Types – Examples include Classes, Interface.
What is Pointer Type?
The pointer in C# language is a variable, it is also known as locator or indicator that points to an address of value that means pointer type variables stores the memory address of another type. We will discuss this concept in detail in a later article.
Built-in Data Types in C#
The built-in Data Types in C# are as follows
- Boolean type – Only true or false
- Integral Types – sbyte, byte, short, ushort, int, uint, long, ulong, char
- Floating Types – float and double
- Decimal Types
- String Type
What is Escape Sequence in C#?
Verbatim Literal is a string with an @ symbol prefix, as in @“Hello”. The Verbatim literals make escape sequences translate as normal printable characters to enhance readability.
Without Verbatim Literal: “C:\\Pranaya\\DotNetTutorials\\Csharp” – Less Readable
With Verbatim Literal: @“C:\Pranaya\ DotNetTutorials\Csharp” – Better Readable
Let’s understand this with an example:
- namespace FirstProgram
- {
- class Program
- {
- static void Main(string[] args)
- {
- // Displaying double quotes in c#
- string Name = "\"Dotnettutorials\"";
- Console.WriteLine(Name);
- // Displaying new line character in c#
- Name = "One\nTwo\nThree";
- Console.WriteLine(Name);
- // Displaying new line character in c#
- Name = "c:\Pranaya\Dotnettutorials\Csharp";
- Console.WriteLine(Name);
- // C# verbatim literal
- Name = @"c:\Pranaya\Dotnettutorials\Csharp";
- Console.WriteLine(Name);
- Console.ReadKey();
- }
- }
- }
OUTPUT:
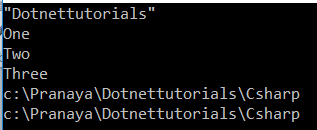
That’s it for today. In the next article, I am going to discuss the Static and Non-Static Member in C# with examples. Here, in this article, I try to explain the Data Types in C# with some examples. I hope you understood the need and use of data types and I would like to have your feedback about this article.
0 comments:
Post a Comment
Note: only a member of this blog may post a comment.