In this article, I am going to discuss the Basic Structure of C# Program using a console application. Please read our previous article before proceeding to this article where we discussed the DOT NET Program Execution Flow in detail. As part of this article, I am going to discuss the following pointers in detail.
- What is C#.NET?
- Advantages of using the .NET Framework from the C# point of view.
- Different Types of applications developed using C#.NET.
- What is the visual studio?
- What is a console application?
- How to Create a console application using the visual studio?
- Example of console application using C#.
- Understanding the basic structure of a C# Program.
Importing section
Namespace Declaration
Class Declaration
Main() method
So, here, first, we will understand what is C#.Net and Visual Studio and what type of applications we can develop using C#.Net. Then we will discuss the basic structure of a C# program using a console application.
What is C#.NET?
- C#.NET is one of the Microsoft programming languages.
- It is the most powerful programming language among all programming languages available in .NET framework because it contains all the features of C++, VB.NET, JAVA and also some additional features.
- C#.NET is a completely object-oriented programming language.
- This programming language is intended to be a simple, modern, general-purpose object-oriented programming language.
Advantages of using the .NET framework from the C# point of view.
- It provides GUI features. Earlier programming languages like C, C++ does not support GUI features but C#.NET will provide complete GUI features. All GUI features are getting from the framework.
- Any database will support and perform the operations. Using ADO.NET technology we can perform the operations with any DB server. ADO.NET is also a part of the framework.
- It prepares WEB applications. Using ASP.NET technology we can implement web applications. ASP.NET is needed language support so we are using either VB or C# as language supporter. ASP.NET is also a part of the framework.
Different Types of applications developed using C#.NET.
- Windows applications
- Web applications
- Console applications
- Class library:
What is the visual studio?
Visual Studio is one of the Microsoft IDE tools. Using this tool we can implement applications with .NET framework. This tool provides some features such as
- Editor
- Compiler
- Interpreter
What is a console application?
- These applications contain a similar user interface to the OS like MS-DOS, UNIX, etc.
- The console application is known as CUI application because in this application we completely work with CUI environment.
- These applications are similar to c or c++ applications.
- Console applications do not provide any GUI facilities like the mouse pointer, colors, buttons, menu bars, etc.
Let’s First Understand the Basic Structure of C# Program using a console application.
- //List of classlibraries /namespaces
- namespace namespacename
- {
- class classname
- {
- static void Main(string[] args)
- {
- //statements
- }
- }
- }
The above process is shown in the below diagram.
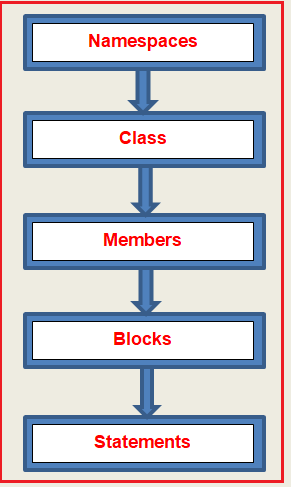
NOTE: C#.NET is a case-sensitive language and every statement in C# should end with a semicolon.
How to Create a console application using the visual studio?
Open visual studio 2015 (You can use any lower or higher version). Go to the new project (File => New => Project) as shown in the below image.
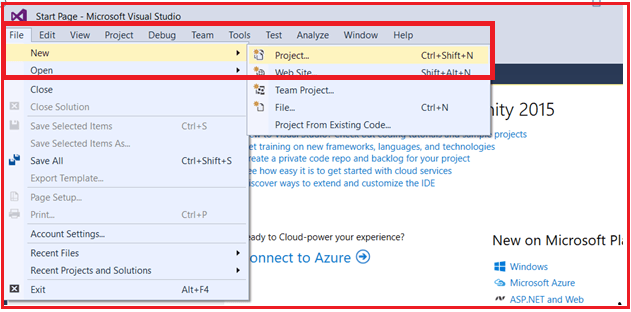
In the next window, select Installed => Templates => Visual C# from the left pane. From the middle Panel, select Console Application. Then specify the application name and location and finally click on the OK button as shown in the below image.
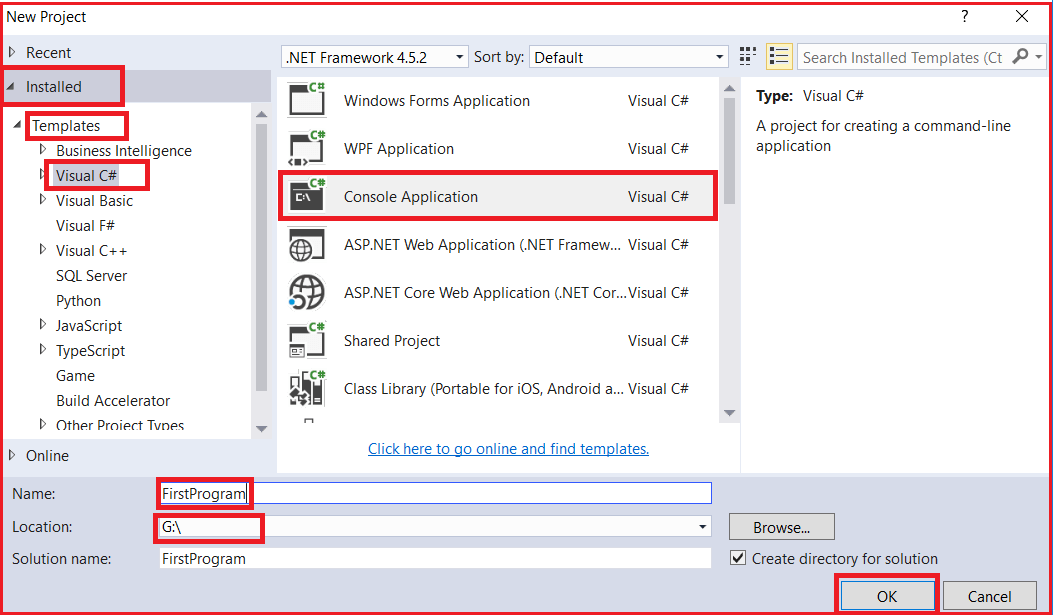
Once you click on the OK button, it will take some time to create the project solution for us.
Let’s create one program to print a welcome message on the console.
Whenever you create a console application, by default the .NET Framework creates a class (i.e. Program class) for us. Let’s modify the Program.cs file as shown below to print a welcome message on the console screen.
- using System;
- namespace FirstProgram
- {
- class Program
- {
- static void Main(string[] args)
- {
- Console.WriteLine("Welcome to C#.NET");
- Console.ReadKey();
- }
- }
- }
Through visual studio whenever we are creating one console application, automatically we are getting four sections as shown in the image below.
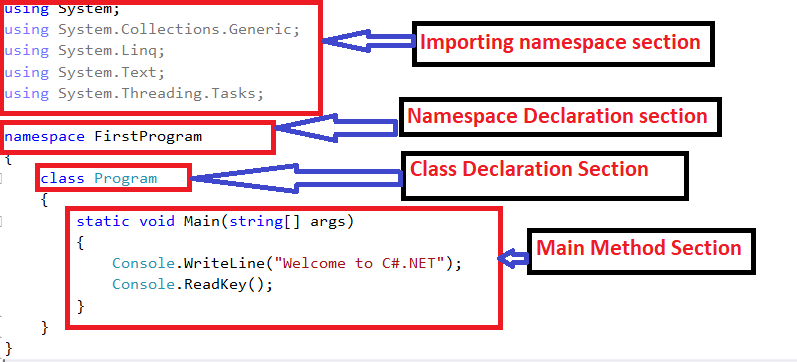
Let’s understand each of these sections in details.
Importing section:
This section contains importing statements that are used to import the BCL (Base Class Libraries). This is similar to the include statements in the C programming language.
Syntax: using namespace;
Example: using System;
Example: using System;
Note: If the required namespace is a member of another namespace we have to specify the parent and child namespaces separated by a dot.
using System.Data;
using System.IO;
using System.IO;
Namespace Declaration:
Here a user-defined namespace is to be declared. In .NET applications, all classes related to the project should be declared inside one namespace.
Syntax:
namespace NamespaceName
{
}
namespace NamespaceName
{
}
Generally, the namespace name will be the same as the project name.
Class Declaration:
This is to declare the start-up class of the project. In every .NET applications like console and windows applications, there should be a start-up class. In this application, the start-up class name is “program”. A startup class is nothing but a class which contains a “Main()” method.
Syntax:
class ClassName
{
}
{
}
Main() method:
The main() method is the starting execution point of the application. When the application is executed the main method will be executed first. This method contains the main logic of the application.
What is using?
Using is a keyword. Using this keyword we can refer to .NET BCL in C# applications.
Note: In .NET the base class libraries are divided into a collection of namespaces. Each namespace contains a set of predefined classes and sub-namespaces. The namespace contains another namespace is called as sub-namespaces.
In the next article, I am going to discuss the Console Class Methods and Properties in detail. Here, in this article, I try to explain the Basic Structure of C# Program step by step with one example. I hope you enjoy this article.
0 comments:
Post a Comment
Note: only a member of this blog may post a comment.